With Azure AD B2B collaboration, we can invite guest users into our tenant to allow them to access M365 apps and other Azure AD SSO integrated apps. There are multiple ways to invite external users into our Azure AD tenant. In this post, I’ll go through what I worked on recently to invite a bunch of external users in to one of the tenants I manage.
Make sure you are connected to Azure AD,
$credential = Get-Credential
Connect-AzureAD -credential $credential
To send an invite to an external user:
$GuestName = Read-Host "Enter name of user being invited"
$GuestEmail = Read-Host "Enter email address of user being invited"
New-AzureADMSInvitation -InvitedUserDisplayName $GuestName -InvitedUserEmailAddress $GuestEmail -SendInvitationMessage $True -InviteRedirectUrl "http://myapps.microsoft.com"
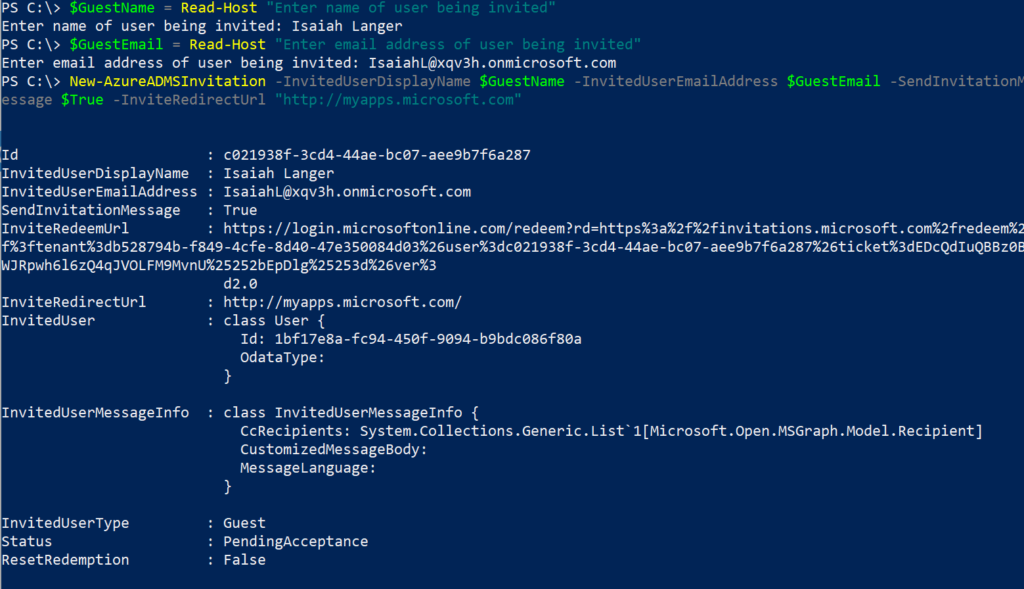
To bulk invite users from a csv file, this below script will work. This script checks to make sure if the user is already invited and also includes a custom message you can include in the invite.
How the csv file looks like,
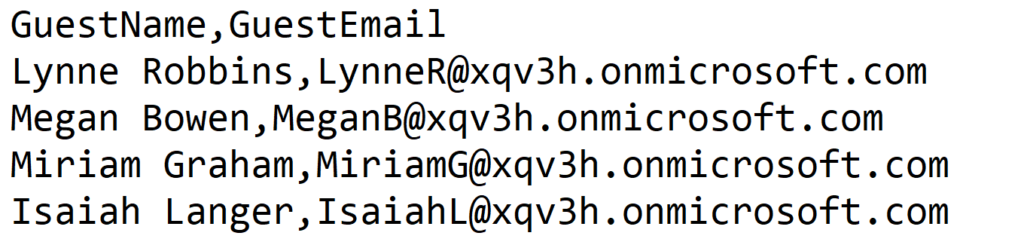
$GuestUsers = Import-csv "C:\tmp\InviteGuestUsers\GuestUsers.csv"
$invitationBody = @"
Hi,
Please accept this invitation to join our organization.
If you have any questions, please contact our helpdesk at
support@internaldomain.com
"@
$invitemessage = @{customizedmessagebody = $invitationBody}
ForEach($GuestUser in $GuestUsers) {
$GuestUserEmail = $GuestUser.GuestEmail
$GuestUserName = $GuestUser.GuestName
if ((Get-AzureADUser -SearchString $GuestUserEmail).Length -le 0) {
$Invite = New-AzureADMSInvitation -InvitedUserDisplayName $GuestUserName -InvitedUserEmailAddress $GuestUserEmail -SendInvitationMessage $True -InvitedUserMessageInfo $invitemessage -InviteRedirectUrl "http://myapps.microsoft.com"
Write-Host "Guest user $GuestUserName invited" -ForegroundColor Green
} else {
$status = Get-AzureADUser -filter "Mail eq '$GuestUserEmail'" | Select -Expandproperty UserState
Write-Host "Guest user $GuestUserName already invited - Guest account exists in tenant and invite status is $status" -ForegroundColor Red
}
}
To list all guest users in the tenant and their invitation acceptance status:
Get-AzureADUser -filter "UserType eq 'Guest'" | Select DisplayName,UserPrincipalName,UserState

or a filter can be applied to determine a specific user,
$guestuser = Read-Host "Enter the guest user email address to check invite status"
Get-AzureADUser -filter "Mail eq '$guestuser'" | Select DisplayName,UserPrincipalName,UserState
Hope this post helped you in external user invites.
Thank you for stopping by. ✌