Azure AD allows granting access to resources by providing access rights to a single user or to an entire Azure AD group. Using groups let the application or the resource owner to assign a set of permissions to all the members of a group. Management rights can be granted to other roles, like example., Helpdesk administrators to add or remove members from the group.
When a group is assigned to an application, only users in the group will have access. Also, if the application exposes role, roles can also be assigned to groups or users.
When I was working on integrating Salesforce with Azure AD for SSO, I needed to assign groups to the roles that Salesforce exposed and I figured I’d document the process I went though here.
Table of Contents
Bulk create Azure AD groups
This section describes how to create multiple groups in Azure AD. This is not needed if your organization already has groups created.
Use below script to create multiple Azure AD groups that are listed in a csv file,
Connect-AzureAD
$groups = import-csv "C:\tmp\AzureAD Groups\groups.csv"
Foreach($group in $groups) {
New-AzureADGroup -DisplayName $group.name -Description $group.description -MailEnabled $false -SecurityEnabled $true -MailNickName "NotSet"
}
csv file input,
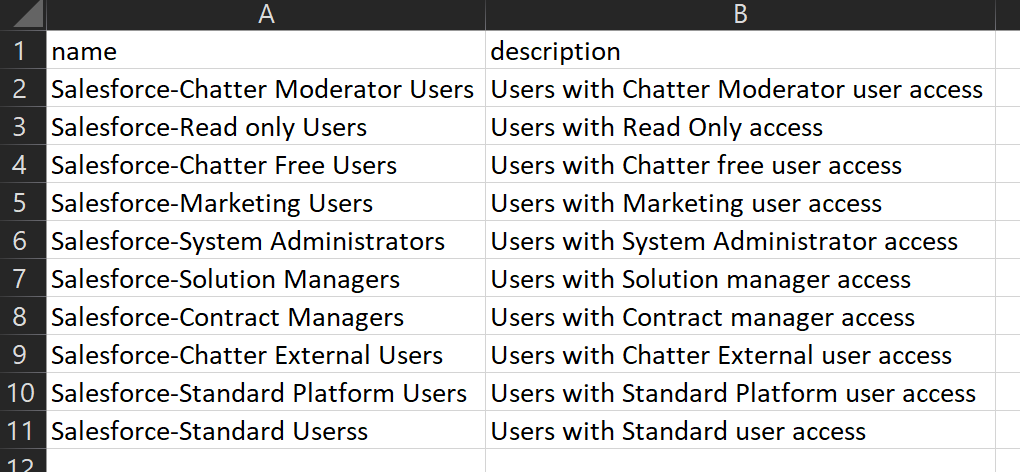
PowerShell output,
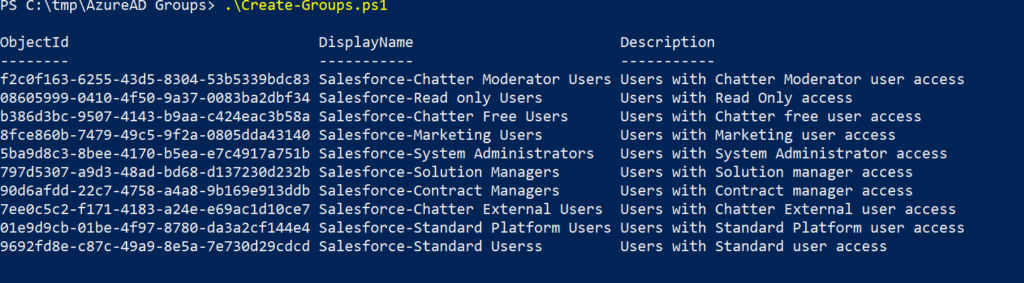
Assign Groups and Users to an app using PowerShell
Assigning groups or users can be done from the Azure AD admin portal by clicking on the Users and groups tab in the application which you are granting access to.
My plan here is to create Azure AD groups that corresponds to the name of the role that Salesforce exposes and then add users to those groups which provides them with appropriate access to the application.
Determine the roles available for the application
To determine the roles that the application exposes, use the cmdlet below.
$appname = Read-Host "Enter your App's Display Name"
$spo = Get-AzureADServicePrincipal -Filter "Displayname eq '$appname'"
$spo.AppRoles | ft DisplayName,IsEnabled,Id
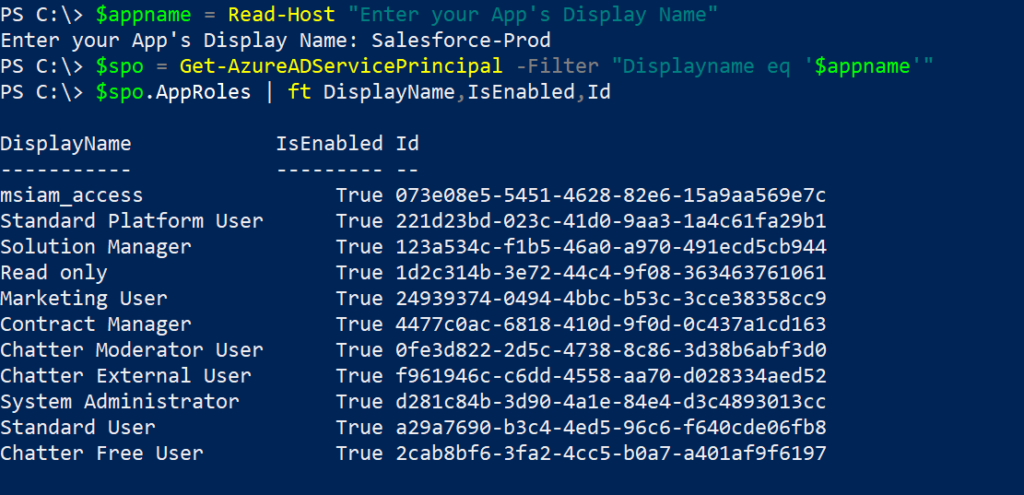
Assign Groups to Roles in Application
Use below script to assign the application’s roles to groups. If you notice the csv file, I’m using the groups created in the previous step to the roles. This way, it is easier to manage. The New-AzureADGroupAppRoleAssignment cmdlet can be used to achieve this.
$appname = Read-Host "Enter your App's Display Name"
$spo = Get-AzureADServicePrincipal -Filter "Displayname eq '$appname'"
$groups = import-csv "C:\tmp\Salesforce_Asgn\groups.csv"
Foreach($group in $groups) {
$id = Get-AzureADGroup -SearchString $group.name
$app_role_name = $group.role
$app_role = $spo.AppRoles | Where-Object { $_.DisplayName -eq $app_role_name }
New-AzureADGroupAppRoleAssignment -ObjectId $id.ObjectId -PrincipalId $id.ObjectId -ResourceId $spo.ObjectId -Id $app_role.Id
}
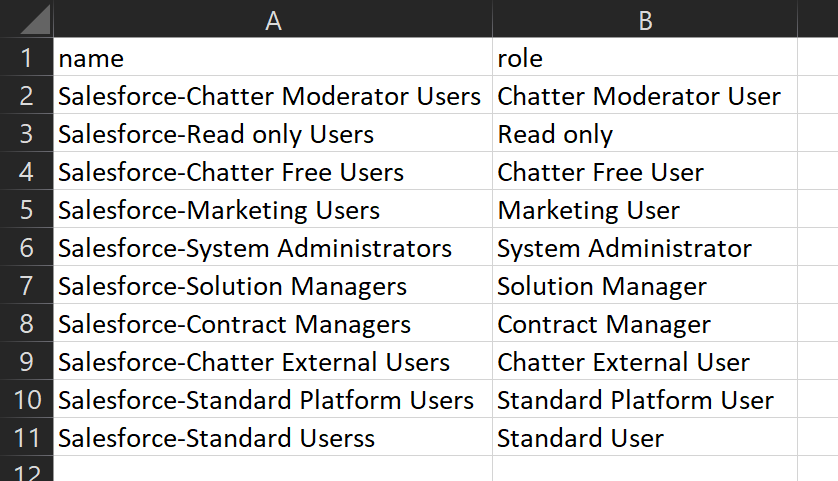
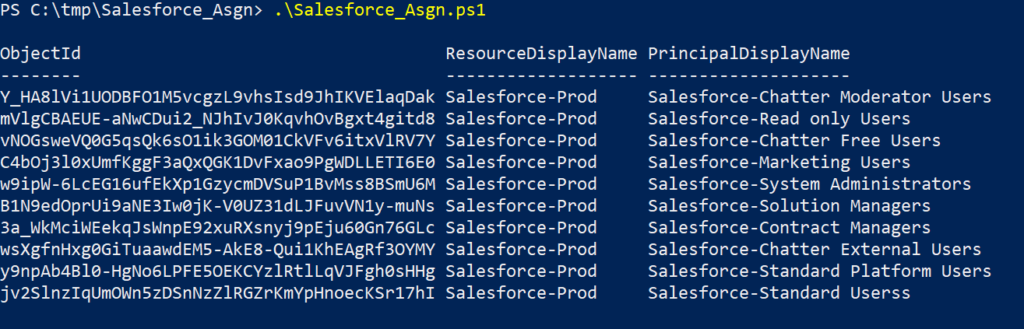
This below is how the application looks like in the Azure AD admin portal after running the above script,
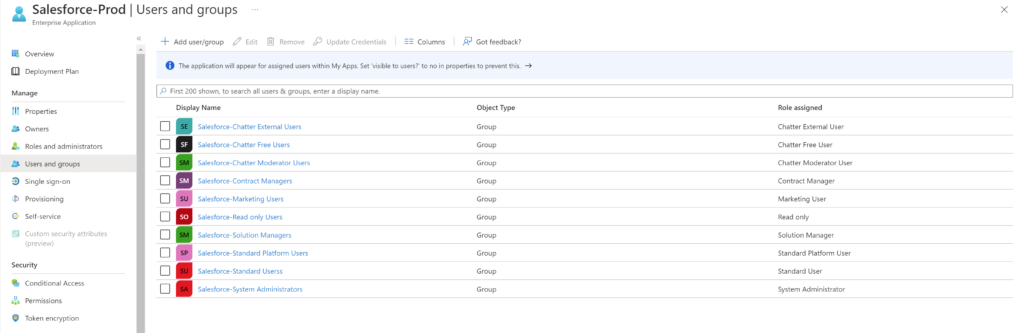
Assign Users to Roles in Application
Use below script to assign the application’s roles to users. This can be achieved using the New-AzureADUserAppRoleAssignment cmdlet. Use the below script,
$appname = Read-Host "Enter your App's Display Name"
$spo = Get-AzureADServicePrincipal -Filter "Displayname eq '$appname'"
$users = import-csv "C:\tmp\Salesforce_Asgn\users.csv"
Foreach($user in $users) {
$id = Get-AzureADUser -ObjectId $user.name
$app_role_name = $user.role
$app_role = $spo.AppRoles | Where-Object { $_.DisplayName -eq $app_role_name }
New-AzureADUserAppRoleAssignment -ObjectId $id.ObjectId -PrincipalId $id.ObjectId -ResourceId $spo.ObjectId -Id $app_role.Id
}
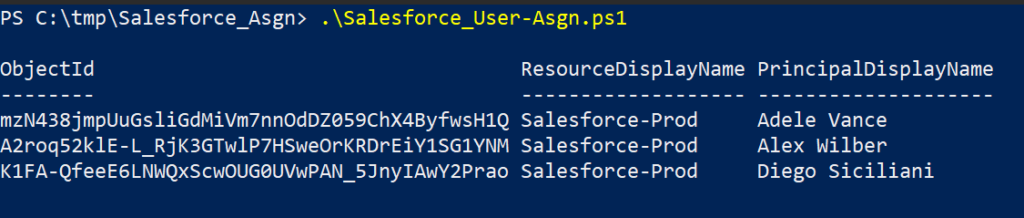
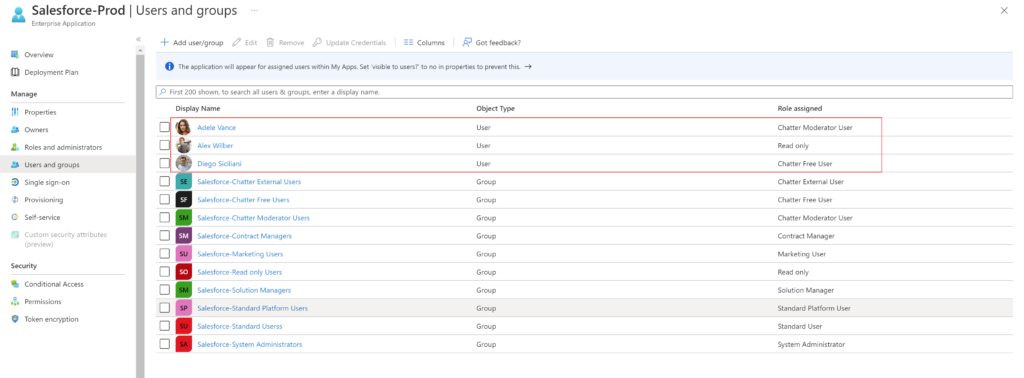
Get all role assignments to an application using PowerShell
Get-AzureADServiceAppRoleAssignment cmdlet can be used to determine all role assignments to an application,
$appname = Read-Host "Enter your App's Display Name"
$spo = Get-AzureADServicePrincipal -Filter "Displayname eq '$appname'"
Get-AzureADServiceAppRoleAssignment -ObjectId $spo.ObjectId -All $true
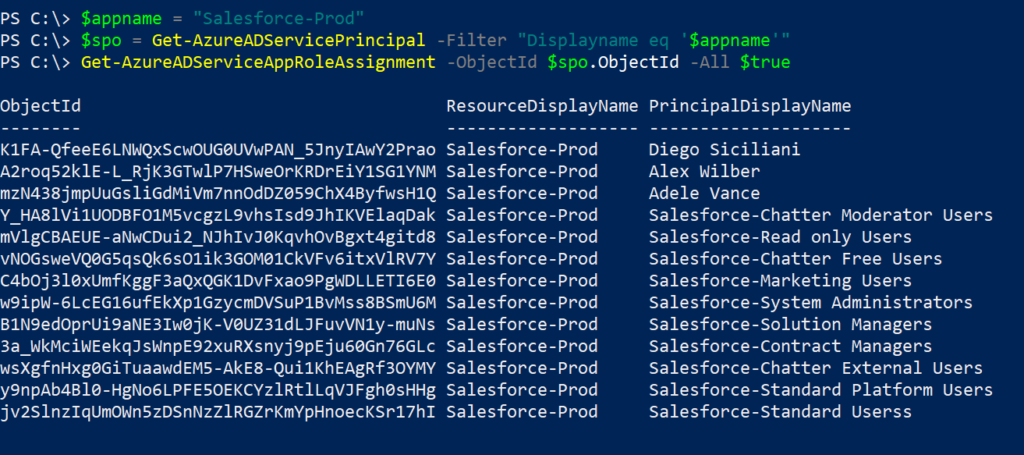
Remove All Groups and Users assigned to an application
To remove all assigned groups and users from an application, Remove-AzureADServiceAppRoleAssignment cmdlet can be used,
$appname = Read-Host "Enter your App's Display Name"
$spo = Get-AzureADServicePrincipal -Filter "Displayname eq '$appname'"
$app_assignments = Get-AzureADServiceAppRoleAssignment -ObjectId $spo.ObjectId -All $true
$app_assignments | ForEach-Object {
if ($_.PrincipalType -eq "user") {
Remove-AzureADUserAppRoleAssignment -ObjectId $_.PrincipalId -AppRoleAssignmentId $_.ObjectId
} elseif ($_.PrincipalType -eq "Group") {
Remove-AzureADGroupAppRoleAssignment -ObjectId $_.PrincipalId -AppRoleAssignmentId $_.ObjectId
}
}
It should go without saying that removing all permissions will disable user’s access to the application. Don’t try this as a first step in a production environment, unless you are absolutely sure of it.
Thank you for stopping by.✌
1 thought on “Azure AD – Assign Groups and Users to an application”