Azure AD security groups can be used to manage member and computer access to shared resources for a group of users. A security group can have users, devices, groups and SPNs as its members. In this post, I’ll go over steps on how to create a Azure AD security group, add users to one group and also bulk import users to one group and multiple groups.
Make sure you are connected to Azure AD,
$credential = Get-Credential
Connect-AzureAD -credential $credential
To create an Azure AD security group:
$GroupName = Read-Host "Enter name for Azure AD group"
New-AzureADGroup -DisplayName $GroupName -MailEnabled $false -SecurityEnabled $true -MailNickName "NotSet"
To create multiple Azure AD security groups:
Note: csv file has name,description as column headers
$groups = import-csv "C:\tmp\AzureAD Groups\groups.csv"
Foreach($group in $groups) {
New-AzureADGroup -DisplayName $group.name -Description $group.description -MailEnabled $false -SecurityEnabled $true -MailNickName "NotSet"
}
To add an user and an owner to an existing group:
$Group = Read-Host "Enter name of Azure AD group"
$User = Read-Host "Enter username of user to be added"
$Owner = Read-Host "Enter username of group owner"
$GroupObj = Get-AzureADGroup -SearchString $Group
$UserObj = Get-AzureADUser -SearchString $User
$OwnerObj = Get-AzureADUser -SearchString $Owner
Add-AzureADGroupMember -ObjectId $GroupObj.ObjectId -RefObjectId $UserObj.ObjectId
Add-AzureADGroupOwner -ObjectId $GroupObj.ObjectId -RefObjectId $OwnerObj.ObjectId
To display members of a security group:
$Group = Read-Host "Enter name of Azure AD group to display members"
$GroupObj = Get-AzureADGroup -SearchString $Group
Get-AzureADGroupMember -ObjectId $GroupObj.ObjectId
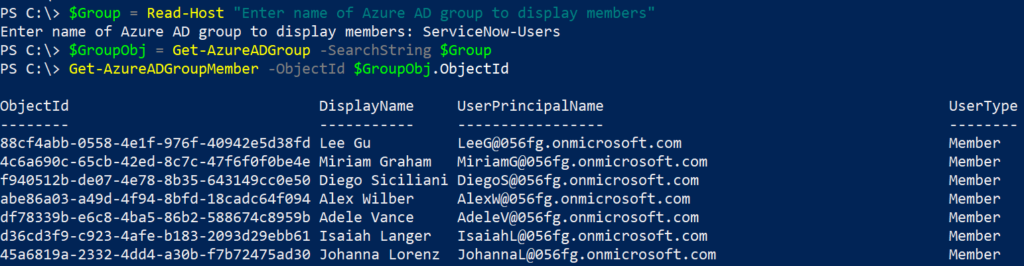
To display owners of a security group:
$Group = Read-Host "Enter name of Azure AD group to display owner(s)"
$GroupObj = Get-AzureADGroup -SearchString $Group
Get-AzureADGroupOwner -ObjectId $GroupObj.ObjectId
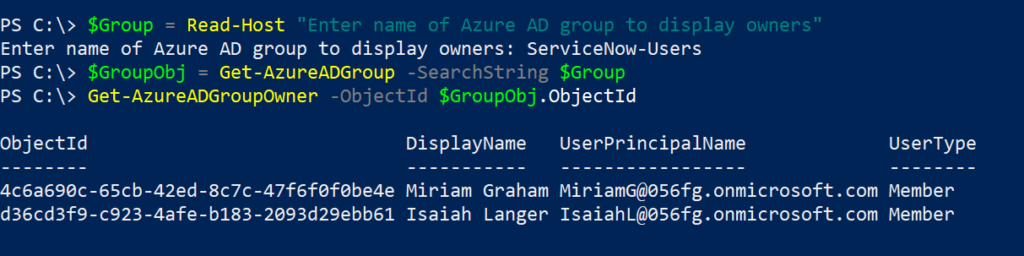
To bulk add multiple users to a specific group:
Note: csv file has UserPrincipalName as column header
$Group = Read-Host "Enter name of Azure AD group to add users"
$GroupObj = Get-AzureADGroup -SearchString $Group
$Members = Import-csv "C:\tmp\GroupMembers.csv"
Foreach($Member in $Members) {
$User = $Member.UserPrincipalName
Write-Progress -Activity "Adding user.." -Status $User
Try {
$UserObj = Get-AzureADUser -SearchString $User
Add-AzureADGroupMember -ObjectId $GroupObj.ObjectId -RefObjectId $UserObj.ObjectId
}
catch {
Write-Host "Error occured while adding $User" -ForegroundColor Red
}
}
To bulk add multiple users to multiple groups. This below script checks to see if the user is already part of the Azure AD group and returns an error.
Note: csv file has UserPrincipalName,Group as column headers
$list = Import-csv "C:\tmp\UsersGroups.csv"
Foreach($entry in $list) {
$User = $entry.UserPrincipalName
$Group = $entry.Group
$UserObj = Get-AzureADUser -SearchString $User
$GroupObj = Get-AzureADGroup -SearchString $Group
$GroupMembers = (Get-AzureADGroupMember -ObjectId $GroupObj.ObjectId).UserPrincipalName
If ($User -notin $GroupMembers) {
Add-AzureADGroupMember -ObjectId $GroupObj.ObjectId -RefObjectId $UserObj.ObjectId
Write-Host "$User added to $Group" -ForegroundColor Green
}
else {
Write-Host "$User already in $Group" -ForegroundColor Red
}
}
Hope this was helpful for you in exploring Azure AD security groups.
Thank you for stopping by. ✌